- Comment.jsx
import React from "react";
const styles = {
wrapper: {
margin: 8,
padding: 8,
display: "flex",
flexDirection: "row",
border: "1px solid grey",
borderRadius: 16,
},
imageContainer: {},
image: {
width: 50,
height: 50,
borderRadius: 25,
},
contentContainer: {
marginLeft: 8,
display: "flex",
flexDirection: "column",
justifyContent: "center",
},
nameText: {
color: "black",
fontSize: 16,
fontWeight: "bold",
},
commentText: {
color: "black",
fontSize: 16,
},
};
function Comment(props) {
return (
<div style={styles.wrapper}>
<div style={styles.imageContainer}>
<img
src="https://upload.wikimedia.org/wikipedia/commons/8/89/Portrait_Placeholder.png"
style={styles.image}
/>
</div>
<div style={styles.contentContainer}>
<span style={styles.nameText}>{props.name}</span>
<span style={styles.commentText}>{props.comment}</span>
</div>
</div>
)
}
export default Comment;
- CommentList.jsx
import React from "react";
import Comment from "./Comment";
const comments = [
{
name:"홍길동",
comment:"안녕하세요, 홍길동입니다.",
},
{
name:"스파이더맨",
comment:"Hello, my name is spiderman",
},
{
name:"코난",
comment:"진실은 언제나 하나",
},
];
function CommentList(props) {
return (
<div>
{comments.map((comment) => {
return (
<Comment name={comment.name} comment={comment.comment} />
);
})}
</div>
);
}
export default CommentList;
- index.js
import React from 'react';
import ReactDOM from 'react-dom/client';
import './index.css';
import App from './App';
import reportWebVitals from './reportWebVitals';
//import Clock from './chapter_04/Clock';
//import { render } from '@testing-library/react';
import CommentList from './chapter_05/CommentList';
const root = ReactDOM.createRoot(document.getElementById('root'));
root.render(
<React.StrictMode>
<CommentList />
</React.StrictMode>,
document.getElementById('root')
);
// If you want to start measuring performance in your app, pass a function
// to log results (for example: reportWebVitals(console.log))
// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitals
reportWebVitals();
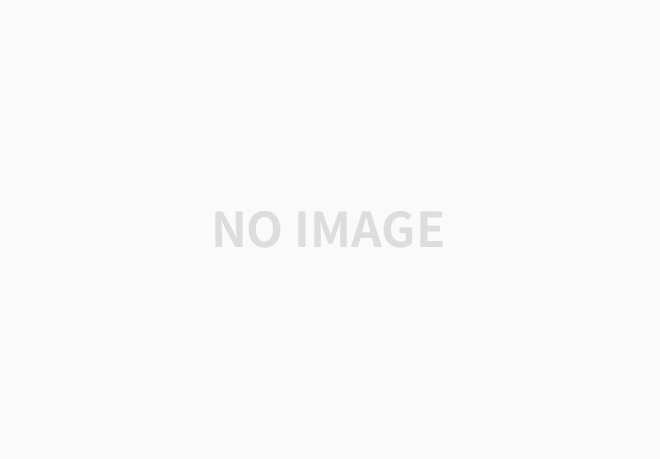
'Front-end > React' 카테고리의 다른 글
[React] 실습 - 시계 만들기 (0) | 2023.05.24 |
---|---|
[React] JSX 정의, 장점, 사용법 (0) | 2023.05.24 |
[React] create-react-app (0) | 2023.05.17 |
[React] HTML 코드에 직접 리액트 연동 (0) | 2023.05.17 |
[React] 리액트의 정의, 장단점 (0) | 2023.05.17 |